The Elf Code
Objective
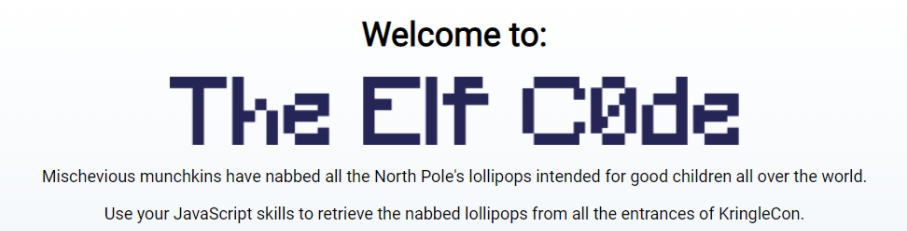
Instead of using traditional game controls, write javascript code to move the character around the game map, collect items, and interact with non-player characters.
Solution
Level 1 - Program the elf to the end goal in no more than 2 lines of code and no more than 2 elf commands.
elf.moveLeft(10)
elf.moveUp(10)
Level 2 - Program the elf to the end goal in no more than 5 lines of code and no more than 5 elf command/function execution statements in your code.
elf.moveLeft(6)
var sum = elf.get_lever(0) + 2
elf.pull_lever(sum)
elf.moveLeft(4)
elf.moveUp(10)
Level 3 - Pick up all the lollipops. Program the elf to the end goal in no more than 4 lines of code and no more than 4 elf command/function execution statements in your code.
elf.moveTo(lollipop[0])
elf.moveTo(lollipop[1])
elf.moveTo(lollipop[2])
elf.moveUp(1)
Level 4 - Program the elf to the end goal in no more than 7 lines of code and no more than 6 elf command/function execution statements in your code.
for (var i = 0; i < 5; i++) {
elf.moveLeft(3)
elf.moveUp(11)
elf.moveLeft(3)
elf.moveDown(11)
}
Level 5 - Program the elf to the end goal in no more than 10 lines of code and no more than 5 elf command/function execution statements in your code..
elf.moveTo(munchkin[0])
var array = elf.ask_munch(0)
var answer = []
array.forEach(function(item) {
if (typeof item === 'number') {
answer.push(item)
}
});
elf.tell_munch(answer)
elf.moveUp(2)
Level 6 - Program the elf to the end goal in no more than 15 lines of code and no more than 7 elf command/function execution statements in your code.
for (var i = 0; i < 4; i++) {
elf.moveTo(lollipop[i])
}
elf.moveTo(lever[0])
var list = elf.get_lever(0)
list.unshift("munchkins rule")
elf.pull_lever(list)
elf.moveDown(3)
elf.moveLeft(6)
elf.moveUp(10)
Level 7 (Bonus Level) - Program the elf to the end goal in no more than 25 lines of code and no more than 10 elf command/function execution statements in your code.
function YourFunctionNameHere(arrays) {
var sum = 0
arrays.forEach(function(array) {
array.forEach(function(value) {
if( typeof(value) == 'number') {
sum += value
}
});
});
return sum
}
var directions = [elf.moveDown, elf.moveLeft, elf.moveUp, elf.moveRight, elf.moveDown, elf.moveLeft, elf.moveUp, elf.moveRight]
for (var i = 0; i < 7; i++) {
directions[i](i + 1)
elf.pull_lever(i)
}
elf.moveRight(8)
elf.moveUp(2)
elf.moveLeft(4)
answer = elf.ask_munch(0)
elf.tell_munch(YourFunctionNameHere)
elf.moveUp(1)
Level 8 (Bonus Level) - Program the elf to the end goal in no more than 40 lines of code and no more than 10 elf command/function execution statements in your code.
function YourFunctionNameHere(a) {
for ( var x=0; x < a.length; x++ ) {
b = a[x]
c = Object.keys(b).find(key => b[key] == 'lollipop')
if (typeof(c) == 'string') {
return c
break
}
}
}
var hohoho = 0
for (var i = 0; i < 6; i++) {
if (i % 2 == 0) {
elf.moveRight((i * 2) + 1)
} else {
elf.moveLeft((i * 2) + 1)
}
hohoho += elf.get_lever(i)
elf.pull_lever(hohoho)
elf.moveUp(2)
}
elf.tell_munch(YourFunctionNameHere)
elf.moveRight(11)