Speaker UNprep
Objective
There are three challenges in this terminal that will: open the Speaker UnPrep room door, turn on the lights, and turn on a vending machine. A lab folder is provided so you can experiment with writable copies of the commands and their configuration files.
Open the door
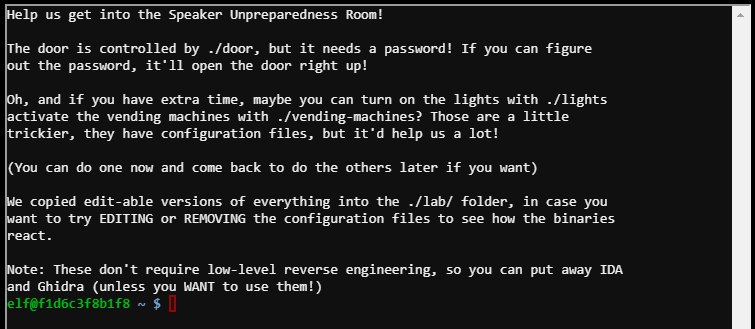
This terminal challenge offers to open the door to the Speaker Unpreparedness Room by providing a password to the door program. Since door is an elf binary executable, you can examine the text strings inside of it to find the password.
$ file door
door: ELF 64-bit LSB shared object, x86-64, version 1 (SYSV), dynamically linked, interpreter /lib64/ld-linux-x86-64.so.2, for GNU/Linux 3.2.0, BuildID[sha1]=4973fb994a1038936fd6111fa1e01d95f052a1dd, stripped
$ strings door | grep pass
/home/elf/doorYou look at the screen. It wants a password. You roll your eyes - the
password is probably stored right in the binary. There's gotta be a
Be sure to finish the challenge in prod: And don't forget, the password is "Op3nTheD00r"
Beep boop invalid password
$ ./door
You look at the screen. It wants a password. You roll your eyes - the
password is probably stored right in the binary. There's gotta be a
tool for this...
What do you enter? > Op3nTheD00r
Checking......
Door opened!
Turn on the lights
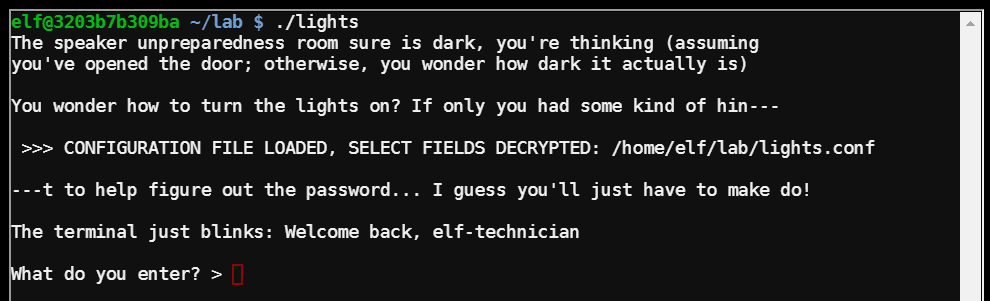
The lights program provides a hint that it decrypts the conf file at runtime. By modifying the lights.conf file and replacing the username with the encrypted password string, the program will provide the unencrypted password at start-up.
$ sed -i 's/elf-technician/E$ed633d885dcb9b2f3f0118361de4d57752712
c27c5316a95d9e5e5b124/' lights.conf
$ ./lights
<...output omitted...>
The terminal just blinks: Welcome back, Computer-TurnLightsOn
Turn on the vending machine
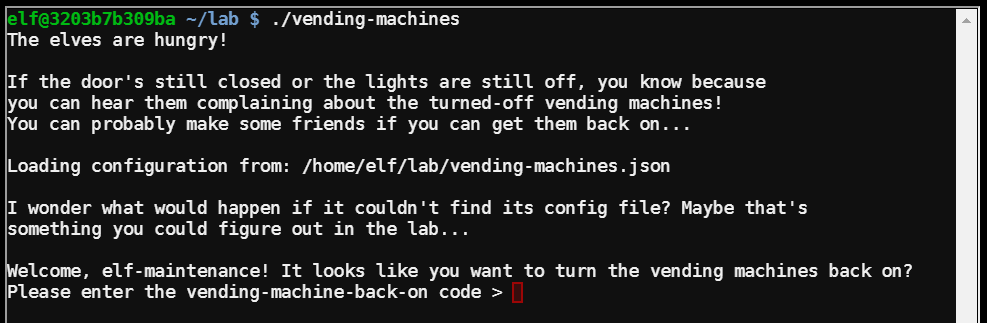
The vending-machines program provides a hint that it behaves differently if its configuration file cannot be found. When you delete the vending-machines.json file and run the program, you're allowed to supply your own username and password, which produces a new json file with an encrypted password.
$ rm vending-machines.json
$ ./vending-machines
<..output omitted..>
ALERT! ALERT! Configuration file is missing! New Configuration File Creator Activated!
Please enter the name > foo
Please enter the password > AAAAAAAAAAAAAAAA
<..output omitted..>
$ cat vending-machines.json
{
"name": "foo",
"password": "XiGRehmwXiGRehmw"
}
Through the process of cryptanalysis, you may be able to determine what encryption is used, and ultimately decrypt the original password. Since you have control over the encryption program and can compare plaintext inputs you choose with the resulting encrypted ciphertext, the technique is known as a chosen plaintext attack.
Start by supplying the program with a long string of A's, and observe that the program encrypts it into the ciphertext "XiGRehmwXiGRehmw". If this were a simple substitution cipher, the letter A would always be replaced with the letter X, however that is not the case here. This ciphertext repeats itself every 8th character, which suggests a polyalphabetic substitution cipher, meaning there are multiple substitutions.
To figure this out, remove the vending_machines.json file again and run the vending-machines program to set a password containing 8 of every character a-z, A-z, and 0-9.
The program generates the following new vending-machines.json file:
$ cat vending-machines.json
{
"name": "q",
"password": "9VbtacpgGUVBfWhPe9ee6EERORLdlwWbwcZQAYue8wIUrf5xkyYSPafTnnUgokAhM0sw4eOCa8okTqy1o63i07r9fm6W7siFqMvusRQJbhE62XDBRjf2h24c1zM5H8XLYfX8vxPy5NAyqmsuA5PnWSbDcZRCdgTNCujcw9NmuGWzmnRAT7OlJK2X7D7acF1EiL5JQAMUUarKCTZaXiGRehmwDqTpKv7fLbn3UP9Wyv09iu8Qhxkr3zCnHYNNLCeOSFJGRBvYPBubpHYVzka18jGrEA24nILqF14D1GnMQKdxFbK363iZBrdjZE8IMJ3ZxlQsZ4Uisdwjup68mSyVX10sI2SHIMBo4gC7VyoGNp9Tg0akvHBEkVH5t4cXy3VpBslfGtSz0PHMxOl0rQKqjDq2KtqoNicv2rDO5LkIpWFLz5zSWJ1YbNtlgophDlgKdTzAYdIdjOx0OoJ6JItvtUjtVXmFSQw4lCgPE6x73ehm9ZFH"
}
With the encrypted password from the original vending-machines.json file, plus the known plaintext used to create the new password, and its resulting ciphertext, you can write a program that decrypts the password using 8 lookup tables on a rotating basis.
#!/usr/bin/env python3
encrypted_password = "LVEdQPpBwr"
known_plaintext = "aaaaaaaabbbbbbbbccccccccddddddddeeeeeeeeffffffffgggggggghhhhhhhhiiiiiiiijjjjjjjjkkkkkkkkllllllllmmmmmmmmnnnnnnnnooooooooppppppppqqqqqqqqrrrrrrrrssssssssttttttttuuuuuuuuvvvvvvvvwwwwwwwwxxxxxxxxyyyyyyyyzzzzzzzzAAAAAAAABBBBBBBBCCCCCCCCDDDDDDDDEEEEEEEEFFFFFFFFGGGGGGGGHHHHHHHHIIIIIIIIJJJJJJJJKKKKKKKKLLLLLLLLMMMMMMMMNNNNNNNNOOOOOOOOPPPPPPPPQQQQQQQQRRRRRRRRSSSSSSSSTTTTTTTTUUUUUUUUVVVVVVVVWWWWWWWWXXXXXXXXYYYYYYYYZZZZZZZZ11111111222222223333333344444444555555556666666677777777888888889999999900000000"
known_ciphertext = "9VbtacpgGUVBfWhPe9ee6EERORLdlwWbwcZQAYue8wIUrf5xkyYSPafTnnUgokAhM0sw4eOCa8okTqy1o63i07r9fm6W7siFqMvusRQJbhE62XDBRjf2h24c1zM5H8XLYfX8vxPy5NAyqmsuA5PnWSbDcZRCdgTNCujcw9NmuGWzmnRAT7OlJK2X7D7acF1EiL5JQAMUUarKCTZaXiGRehmwDqTpKv7fLbn3UP9Wyv09iu8Qhxkr3zCnHYNNLCeOSFJGRBvYPBubpHYVzka18jGrEA24nILqF14D1GnMQKdxFbK363iZBrdjZE8IMJ3ZxlQsZ4Uisdwjup68mSyVX10sI2SHIMBo4gC7VyoGNp9Tg0akvHBEkVH5t4cXy3VpBslfGtSz0PHMxOl0rQKqjDq2KtqoNicv2rDO5LkIpWFLz5zSWJ1YbNtlgophDlgKdTzAYdIdjOx0OoJ6JItvtUjtVXmFSQw4lCgPE6x73ehm9ZFH"
lookup_tables = []
keysize = 8
c = 0
for n in range(0,keysize):
lookup_table = {}
for i in range(n,len(known_plaintext),keysize):
lookup_table[known_ciphertext[i]] = known_plaintext[i]
lookup_tables.append(dict(lookup_table))
for i in range (0,len(encrypted_password)):
print(lookup_tables[c].get(encrypted_password[i]), end="")
if c==7:
c = 0
else:
c+=1
print("")
Run the program for the answer.
$ ./decrypt.py
CandyCane1